The Computing Tutor's Four Rules for Writing Functions or Procedures
- TheComputingTutor
- Sep 6, 2018
- 3 min read
As someone studying A level computer science or BTEC IT or Computing, at some point you will need to write a function, method, procedure or sub-routine. They are all essentially the same thing - they are just called different names in different languages. For example, in C# - everything is a function - they are defined by their return type - a VOID function brings back nothing to the calling program, where as RETURN function brings something back. In VB and VB.NET you have a METHOD which goes away and does something, and you have a PROCEDURE that goes away and brings something back.
Same thing, different names.
However, in all languages the way you structure them is the same, and I'm going to talk you through it.
Lets take a very simple function - it will prompt a user to enter two numbers, it will add them together and then display the result.
The output should look like this:
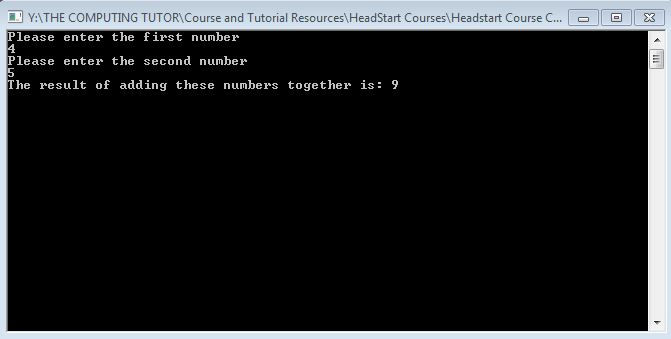
OK the logic of our code should be:
output to the user
store the input as a string
convert the strings to numbers
add the two numbers together
output the result
OK, all pretty simple, right?
well when we start to break it down, writing this simple function contains all the rules for pretty much every function on the planet. I call them Meaney's Rules and they will help you put your functions together.
RULE NUMBER 1 - DECLARE YOUR VARIABLES!
All you variables should be declared at the start of your function. It keeps things simple and you know exactly what's in your function.
You will need a variable to store each piece of user input, you will need variables to store the results of any calculation, and you will need variables to store any data conversion. Here we are changing strings to numbers.
Here are the variables you will need in the simple calculator function in C#
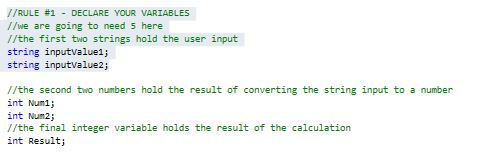
RULE NUMBER 2 - HANDLE THE INPUT!
You will need to output a message to the user and then store all the input as a string.
If you have a function with parameters, you might want to capture these and store the data in a temporary local variable, either way - you will need to deal with data coming into your Function.

RULE NUMBER 3 - DO THE MATHS!
Every function on the planet exists to do some kind of job that you want to use again and again. This is why we create functions.
Usually the function will involve some form of calculation, for example doing some complex maths, or processing information from a data structure - for example a binary tree, linked list or array. In this example, we are simply converting a string to a number and adding them together, but the principle holds true.
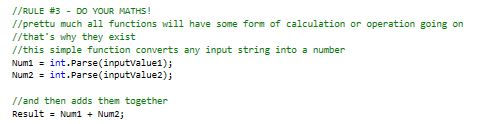
RULE NUMBER 4 - OUTPUT TO THE USER!
At some point your function will finish and you will output a result. Your output could be displaying a result to a user, returning some value to your main program, or saving data to a file or database. Either way, there is some end point to your Function, even if you have defined it as VOID. All of these could be considered a 'user' of your function: a human being, another program, a database or a file.
In this case we are simple displaying the results of the calculation to a human user.

CONCLUSION
If you can remember these rules, you can't really go wrong. Don't worry if you don't identify all your variables at a first go, but by putting them all at the top, you can comment your code and show other users why you have had to add new variables, also by having them all in one place you know where to look to find them, rather than having them scattered throughout your code.
I hope you've found this post useful, and here is the complete source code for the function.
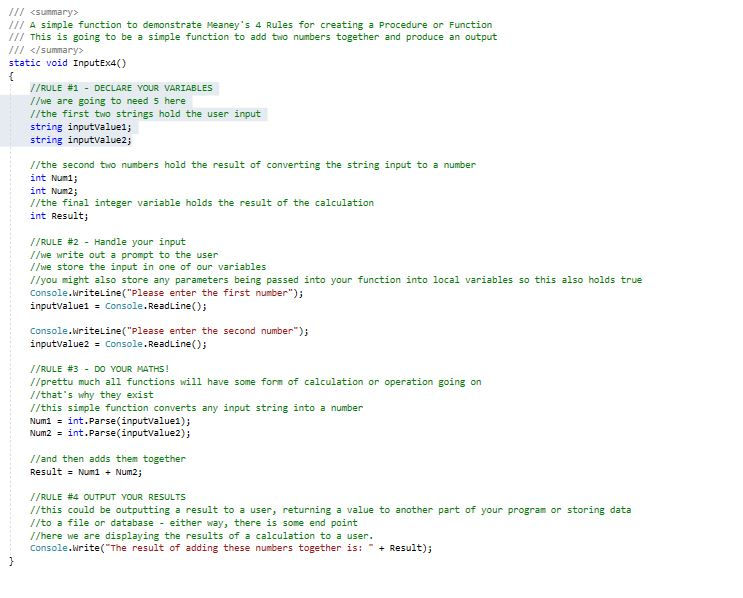
Comments